May 2nd, 2007 — Silverlight
Prepare yourself for some interesting Silverlight posts in the upcoming weeks, I fell in love with the new Microsoft Technology and would like to quote the following statement:
Nik (a long-time developer) was most impressed by how small Silverlight is (4 MB) and how fast it is (it blows away native Javascript routines – without exaggeration, Ajax looks like a bicycle next to a Ferrari when compared to Silverlight).
I agree, I hope Microsoft is promoting it right and will establish Silverlight as a real Flash enemy in the upcoming months. Samples, Tutorials and of course more information on Silverlight will be posted soon. As of now Silverlight Gold is scheduled for summer 2007.
March 29th, 2007 — ASP.NET, WPF
Lester made a sweet WPF/E video carousel which looks pretty nice:
You can download the code on his blog: WPF/E video carousel
March 27th, 2007 — ASP.NET
There are several ways to show who is currently online within your ASP.NET Application if you use the build in Membership Provider. I tested a few on performance and accuary which lead me to the following conclusion:
Using a stored procedure on LastActivityDate is the winner.
Here is how I did it, create this stored procedure:
SET ANSI_NULLS ON
GO
SET QUOTED_IDENTIFIER ON
GO
— =============================================
— Author: Andreas Kraus
— Create date: 2007-03-27
— Description: Who Is Online Box
— =============================================
CREATE PROCEDURE [dbo].[xx_WhoIsOnline]
@TimeWindow DATETIME
AS
BEGIN
— SET NOCOUNT ON added to prevent extra result sets from
— interfering with SELECT statements.
SET NOCOUNT ON;
— Insert statements for procedure here
SELECT UserName FROM aspnet_Users
WHERE IsAnonymous = ‘FALSE’ AND LastActivityDate > @TimeWindow
END
GO
and create a custom ASP.NET Control featuring this code:
18 cn = new SqlConnection(System.Web.Configuration.WebConfigurationManager.ConnectionStrings[“LocalSqlServer”].ConnectionString);
19
20 SqlCommand cmd = new SqlCommand();
21 SqlDataReader dr;
22
23 DateTime TimeWindow = DateTime.Now;
24 TimeSpan TimeSpanWindow = new TimeSpan(0, 10, 0);
25 TimeWindow = TimeWindow.Subtract(TimeSpanWindow);
26
27 cmd.Connection = cn;
28 cmd.CommandText = “battle_WhoIsOnline”;
29 cmd.Parameters.AddWithValue(“@TimeWindow”, TimeWindow);
30 cmd.CommandType = System.Data.CommandType.StoredProcedure;
31
32 cn.Open();
33 dr = cmd.ExecuteReader();
34
35 System.Text.StringBuilder sb = new System.Text.StringBuilder();
36 while (dr.Read())
37 {
38 sb.Append(dr[“UserName”].ToString());
39 }
40 dr.Close();
41 cn.Close();
42
43 if (sb.Length > 0)
44 {
45 sb.Remove(sb.Length – 2, 2);
46 }
47
48 Literal1.Text = sb.ToString();
Include the control on any .aspx page and you are set.
March 9th, 2007 — ASP.NET
We recently dropped one of our commercial libraries, the Rebex.Net.Mail Tool. Instead we are using the build in System.NET.Mail Class from ASP.NET 2.0. In ASP.NET 1.1 you were able to use the System.Web.Mail Class and you were able to send mail via GMail by adding those filters:
ASP.NET v1.1:
SmtpClient smtp = new SmtpClient();
smtp.Host = "smtp.gmail.com";
// - smtp.gmail.com use smtp authentication
mailMsg.Filters.Add("http://schemas.microsoft.com/cdo/configuration/smtpauthenticate", "1");
mailMsg.Filters.Add("http://schemas.microsoft.com/cdo/configuration/sendusername", "xx");
mailMsg.Filters.Add("http://schemas.microsoft.com/cdo/configuration/sendpassword", "xx");
// - smtp.gmail.com use port 465 or 587
mailMsg.FiltersAdd("http://schemas.microsoft.com/cdo/configuration/smtpserverport", "465");
// - smtp.gmail.com use STARTTLS (some call this SSL)
mailMsg.Filters.Add("http://schemas.microsoft.com/cdo/configuration/smtpusessl", "true");
// try to send Mail
As I switched to the new ASP.NET 2.0 System.Net.Mail class the filters function was gone. Important: mailMsg.Headers.Add in the new class is not the equivalent class to that Filters.Add method, that’s what I thought, too, in the beginning.
In fact it got much easier now, but totally different:
ASP.NET v2.0:
// Smtp configuration
SmtpClient smtp = new SmtpClient();
smtp.Host = "smtp.gmail.com";
smtp.Credentials = new System.Net.NetworkCredential("xx", "xx");
smtp.EnableSsl = true;
I’d love to have more specific documentations on those changes, but thanks to Microsoft for making our code smaller once again ;).
February 10th, 2007 — ASP.NET
In case you are using the ASP.NET Membership Provider you probably noticed that LastActivityDate doesn’t update correctly whenever someone checked Remember me on his Login. I got a site running with a Cookie timeout of 10080 seconds, a pretty long time. I need to have an accurate LastActivityDate because I want to display whenever a user has actually been active.
So what now? I want to stick to my cookie timeout value and need to update the LastActivityDate manually. Here’s my approach (I love stored procedures).
First create a stored Procedure:
31 set ANSI_NULLS ON
32 set QUOTED_IDENTIFIER OFF
33 GO
34 — =============================================
35 — Author: Andreas Kraus (http:/www.andreas-kraus.net/blog)
36 — Create date: 2007-02-10
37 — Description: Updates LastActivityDate
38 — =============================================
39 ALTER PROCEDURE [dbo].[aspnet_Membership_UpdateLastActivityDate]
40 @UserName nvarchar(256),
41 @LastActivityDate datetime
42
43 AS
44 BEGIN
45 IF (@UserName IS NULL)
46 RETURN(1)
47
48 IF (@LastActivityDate IS NULL)
49 RETURN(1)
50
51 UPDATE dbo.aspnet_Users WITH (ROWLOCK)
52 SET
53 LastActivityDate = @LastActivityDate
54 WHERE
55 @UserName = UserName
56 END
Then use this code in your global.asax by hitting the Application_AuthenticateRequest Event:
31 void Application_AuthenticateRequest(object sender, EventArgs e)
32 {
33 if (User.Identity.IsAuthenticated)
34 {
35 System.Data.SqlClient.SqlConnection cn = new System.Data.SqlClient.SqlConnection(System.Web.Configuration.WebConfigurationManager.ConnectionStrings[“LocalSqlServer”].ConnectionString);
36 System.Data.SqlClient.SqlCommand cmd = new System.Data.SqlClient.SqlCommand();
37 cmd.Connection = cn;
38 cmd.CommandText = “aspnet_Membership_UpdateLastActivityDate”;
39 cmd.Parameters.AddWithValue(“@UserName “, User.Identity.Name);
40 cmd.Parameters.AddWithValue(“@LastActivityDate”, DateTime.Now);
41 cmd.CommandType = System.Data.CommandType.StoredProcedure;
42 cn.Open();
43 cmd.ExecuteNonQuery();
44 cn.Close();
45 }
46 }
That’s it, now you have a most accurate LastActivityDate value to work with.
February 1st, 2007 — Administration
I bought a new PC for Vista this month. Here’s what I got:
- CPU Intel Core2 Duo E6600 2×2.4GHz TRAY 4MB
- ASUS P5N32-E SLI nForce680i SLI
- 2x 2048MB Corsair PC2-800 CL5 KIT TWIN2X2048
- Coolermaster Mystique RC631 Black ALU o.N
- 500W Enermax Liberty ELT500AWT
- Zalman CNPS 9500 AT
- 2x WD SE16 250GB WD2500KS 7200U/m 16MB
- ASUS (R) 8800GTS 640MB 2xDVI/TV
- Creative (B) X-Fi Xtreme Gamer
I put everything together, started up the PC and was introduced to a freezing/reboot hell. At first it looked like the RAM didn’t work that well with the motherboard, so I changed them. Thing’s were still not stable at all. I upgraded the bios to the latest beta bios (successfully) and BAWM.. that was the end of it. Just a black screen, no booting up anymore. Lots of people are reporting that they have got big problems with nForce680i Chipsets. I’ve sent the board back for a repair now.
In the meantime I got myself the Abit AB9 QuadGT with Intel Chipset. I put everything together yesterday and it’s running very smooth without ANY problems. Intel is the way to go.
nForce is really bogus, I wouldn’t recommend it anymore. Nvidia does great gfx cards but the mainboard chipsets are just horrible.
However, finally I’m setup with Vista, .NET 3.0 and all the other new shiny stuff 🙂
January 14th, 2007 — Personal
I will be in the USA for one week, flying on Monday from Frankfurt/Germany to Los Angeles. The NAMM Show 2007 takes place in Anaheim, I’ll post some impressions when I got back!
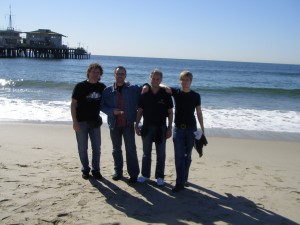
Side node: If you installed .NET 3.0 already and use my Deployment Tool, make sure you download the latest bits, I have set the Compiling Version to the most current 2.x .NET Framework Version so it doesn’t choose 3.0 automatically.
Cheers,
Andreas
December 18th, 2006 — ASP.NET
Version 1.9 adds a little requested feature to my ASP.NET Deployment Tool. Whenever you hit Deploy Local a file called compilerlog.txt will be created now in the Application Folder which holds the compiler output.
So if you got lots of warnings and errors popping up it’s easier to investigate them by looking into compilerlog.txt. Of course using Visual Studio is still best for investigation but it appeared that many people do small fixes without firing up VS once again.
“Bin” is checked by default now: It only copies the content of the bin directory when you hit Copy to FTP.
Short overview for people who don’t know it yet:
An avanced and robust Deployment Tool for precompiling your ASP.NET Websites! Pre-Compilation gives your site a performance boost and secures it.
Precompile and deploy your Website: Specific Error Panel, FTP Support, Deploy to Network, Merge Assemblies, easy to use.
Download it here: ASP.NET Deployment Tool v1.9 (I didn’t update the version number on that page yet, don’t mind it, it’s v1.9)
Edit: I set the background color of the Toolstrip to a static color as many people use black for their desktops 🙂
December 15th, 2006 — Microsoft, Personal
I wanted to do this a long time ago already, now, finally, I finished redoing it. Hope you like it 🙂
ASP.NET AJAX RC1 has been released, the latest release before the fully supported final version
And Visual Studio 2005 SP1 Final is out now: VS 2005 SP1 Final
Enjoy 😉
December 12th, 2006 — Administration
This sometimes happen if you are going to install eaccelerator 0.9.5 for PHP optimizing. We just received our new dedicated server with debian sarge for our vBulletin forum and I’m optimizing it at the moment. There are many results in google for this error, however, none of them helped me.
What actually did help me was this:
apt-get install php5-dev
hth, one of my few non-windows posts 😉
December 7th, 2006 — Administration
I just came up with an idea for our mails at work. We’re battleing spam every day and received more than 23 mio spam mails for 2006 so far. Good spam filters are most of the time very expensive.
What we did now is.. setting a gmail account in between:
One catchall address on our mailserver -> mails are getting forwarded to gmail -> gmail forwards the mails back to our postmaster address -> our local mailserver routes the mails to the proper persons
There you go, you probably can’t get a better spam filter for free as the one from Google.
PS: We don’t care about delivering our mail through Google but it’s eventually not that good for Yahoo or MSN employees 😉
December 3rd, 2006 — Windows
Heads up, Microsoft released a new Remote Desktop for WinXP and Windows 2003 Server which also adds better support for Windows Vista.
Some of the new features:
- Network Level Authentication
- Server Authentication
- Plug and Play redirection
- TS Gateway support
- Monitor Spanning
- 32-bit color and font smoothing
Download for:
– WinXP
– Win2k3 Server
November 22nd, 2006 — Reviews
Sponsored Post: What exactly is the CsvReader?
Csv Reader is an extremely fast and stable .Net class for stream based parsing of virtually any commonly found delimited data format, CSV files, tab delimited files, etc. It’s usable from C#, VB.Net, ASP.Net, or any other .Net language. It’s compatible with the 1.0 .Net framework, 1.1, 2.0, and even the .Net Compact Framework. The methods are designed for ease of use, while the inner architecture is designed purely for speed and efficiency. Parsing is done using the de facto standard CSV file specifications. It handles quoted fields, delimiters in the data, and even data that spans across multiple lines. This gives you the ability to open csv files, edit csv files, and save csv files all directly from code.
I downloaded the .NET Demo and had been impressed by its easy usage. Here is a screenshot of the demo:
You see the original CSV file on the top left side, the XML parsing on the top right side and a DataGrid attached to the CSV file on the bottom of the screen. The processing time is incredible fast as shown here:
CsvReader cleary wins with less than 5 seconds. The coding is really easy, let’s look at the code sample for the textbox and DataGrid of the screenshot above:
175 using (StreamReader reader = new StreamReader(“../../products.csv”))
176 {
177 this.textBox1.Text = reader.ReadToEnd();
178 }
179
180 using (CsvReader reader = new CsvReader(“../../products.csv”))
181 {
182 this.dataGrid1.DataSource = reader.ReadToEnd();
183 }
That’s all, it’s really that simple. With the included samples you can start using CsvReader immediately and implent it in existing applications in just a few seconds.
Bruce recently released CsvReader v2.0 which also enables you to insert data directly into SQL Server which is a great addition to the existing features.
Check out the CsvReader website, it’s the way to go for dealing with csv files. You get 25% off if you order it before November 24th!
November 13th, 2006 — ASP.NET
I’ve dealt with custom ASP.NET Paging lately and wanted to share the fastest possible way to do that with you if you don’t use ADO.NET or any ASP.NET Controls.
Create a stored Procedure like this:
CREATE PROCEDURE [dbo].[usp_PageResults_NAI]
(
@startRowIndex int,
@maximumRows int
)
AS
DECLARE @first_id int, @startRow int
— A check can be added to make sure @startRowIndex isn’t > count(1)
— from employees before doing any actual work unless it is guaranteed
— the caller won’t do that
— Get the first employeeID for our page of records
SET ROWCOUNT @startRowIndex
SELECT @first_id = employeeID FROM employees ORDER BY employeeid
— Now, set the row count to MaximumRows and get
— all records >= @first_id
SET ROWCOUNT @maximumRows
SELECT e.*, d.name as DepartmentName
FROM employees e
INNER JOIN Departments D ON
e.DepartmentID = d.DepartmentID
WHERE employeeid >= @first_id
ORDER BY e.EmployeeID
SET ROWCOUNT 0
GO
And in ASP.NET you execute a DataReader against this piece of code:
42 string SQL = “pics_Paging”;
43
44 cmd.Connection = cn;
45 cmd.CommandText = SQL;
46 cmd.Parameters.AddWithValue(“@startRowIndex”, 6);
47 cmd.Parameters.AddWithValue(“@maximumRows”, 4);
48 cmd.CommandType = System.Data.CommandType.StoredProcedure;
That’s all and it’s pretty speedy, thanks to 4GuysFromRolla.com for the input! All you have to do now is to create the logic for startRowIndex and maximumRows which is a piece of cake 😉
November 9th, 2006 — ASP.NET
Hosting in a basic configuration can be a developer’s pain when it comes to file operations. Usually the webspace doesn’t allow files to be created which has a good reason in that configuration, though.
A very smart way to deal with that is to use Isolated Storages. That way all the files are saved in a virtual storage which also means that you can control the access level which is pretending conflicts with other applications. That’s how it could look like:
176 // Filestore init
177 IsolatedStorageFile isoStore = IsolatedStoargeFile.GetStore(IsolatedStorageScope.Assembly | IsolatedStorageScope.User, null, null);
178
179 // Create dir
180 isoStore.CreateDirectory(“myAppData”);
181 isoStore.CreateDirectory(“myAppData/settings”);
182
183 // Write File into Store
184 IsolatedStoargeFileStream isoStream1 = new IsolatedStorageFileStream(“myAppdData/hello.txt”, System.IO.FileMode.Create, isoStore);
185 byte[] content = Encoding.UTF8.GetBytes(“Hello!”);
186 isoStream1.Write(content, 0, content.Length);
187
188 // Read file
189 IsolatedStorageFileStream isoStream2 = new IsolatedStorageFileStream(“myAppData/hello.txt”, System.IO.FileMode.Open, isoStore);
190 byte[] content2 = new byte[isoStream2.Length];
191 isoStream2.Read(content2, 0, content2.Length);
192 isoStream2.Close();
193
194 Response.Write(Encoding.UTF8.GetString(content2));
195
196 // delete store
197 isoStore.Remove();
That’s all, a pretty clean approach for dealing with files in ASP.NET due to hosting restrictions. Nevertheless it’s also interesting to use in Desktop Applications due to the customized privileges for that storage.